We all know that we can solve any quadratic equation using quadratic formula which is:
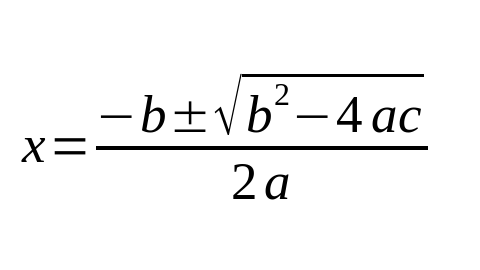
But try to solve this using quadratic formula:
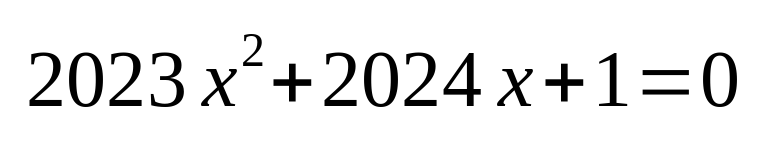
Of course numbers are big and makes calculation very hard but I wanna show you two tricks to solve these equations very fast!
if a+b+c=0, one root is 1 and another is c/a
if a+c=b, one root -1 and another is -c/a
In this equation we see that a+c is b, 2023+1=2024 so one root is -1 and another is -1/2023, so fast right?
but where does these tricks come from? well we need to know one thing, sum of two roots is -b/a:

As you can see the deltas are canceled and we are left with -b/a
now consider a+b+c=0
add -b to whole equation and devide it by a:
a+c=-b
a/a + c/a = -b/a
1 + c/a = -b/a
intresting, we can see the -b/a which is sum of the roots so one root is 1 and another is c/a
now consider a+c=b
multiply -1 to whole equation and divid it by a:
-a-c=-b
-a/a - c/a = -b/a
-1 - c/a = -b/a
again we see the -b/a, so one root is -1 and another is -c/a
I have made an app in java so you can calculate quadratic equations using it:
import java.util.InputMismatchException;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
var main = new Main();
final var scanner = new Scanner(System.in);
while (true) {
System.out.println("Enter e to exit or c to continue");
if (scanner.next().equals("e")) {
scanner.close();
break;
}
try {
System.out.println("Enter a, b and c of the equation: ");
final var a = scanner.nextDouble();
final var b = scanner.nextDouble();
final var c = scanner.nextDouble();
main.solveEquation(a, b, c);
}catch (InputMismatchException e) {
System.out.println("Input is invalid");
scanner.next();
}
}
}
public void solveEquation(double a, double b, double c) {
final var delta = Math.pow(b, 2) - (4*a*c);
if (delta < 0) {
System.out.println("Equation has imaginary results");
final var x1 = String.format("(%s + %si)/%s", -b, Math.sqrt(Math.abs(delta)), 2 * a);
final var x2 = String.format("(%s - %si)/%s", -b, Math.sqrt(Math.abs(delta)), 2 * a);
System.out.printf("x1: %s\nx2: %s\n", x1, x2);
return;
}
final var x1 = (-b + Math.sqrt(delta)) / (2*a);
final var x2 = (-b - Math.sqrt(delta)) / (2*a);
System.out.printf("x1: %s\nx2: %s\n", x1, x2);
}
}